How to deploy python on nginx
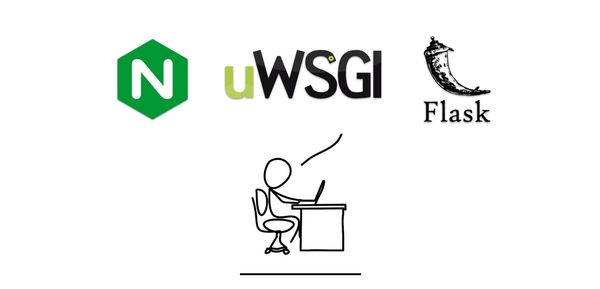
steps to deploy python on nginx
25 Sep 2024
pythonnginx
In this article, I want to share how to deploy applications from Python using Nginx.
1. Install required packages
sudo apt update
sudo apt install python3 python3-pip python3-venv supervisor nginx certbot python3-certbot-nginx
2. Prepare Python Application
- Create Project Directory
mkdir /var/www/app/project
cd /var/www/app/project
- Create virtual environment
python3 -m venv myprojectenv
source myprojectenv/bin/activate
- Install Python libraries used
pip install flask
- Create a simple application (example named app,py)
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, brother pimen!"
if __name__ == '__main__':
app.run()
3. Run Your Application with Gunicorn
- install Gunicorn
pip install gunicorn
- Run the application
gunicorn --bind 0.0.0.0:8000 app:app
by default Gunicorn uses port 8000. you can test the application by visiting http://your_server_ip_address:8000.
4. Nginx Configuration
Create a configuration file
sudo nano /etc/nginx/sites-available/myproject
Add the following configuration
server {
listen 80;
server_name domain_name.com; #replace with ip address or domain
location / {
proxy_pass http://127.0.0.1:8000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
}
}
Activate the configuration that has been created
sudo ln -s /etc/nginx/sites-available/myproject /etc/nginx/sites-enabled
Test the configuration that has been created
sudo nginx -t
Restart service nginx
sudo systemctl restart nginx
5. Supervisor Configuration
Create a configuration file
sudo nano /etc/supervisor/conf.d/myproject.conf
Add the following configuration
[program:myproject]
command=/var/www/app/myprojectenv/bin/gunicorn -w 4 -b 0.0.0.0:8000 app:app
directory=/var/www/app/project
user=your_username
autostart=true
autorestart=true
stopasgroup=true
killasgroup=true
Restart service supervisor
sudo supervisorctl reload sudo supervisorctl status
6. Install ssl
sudo certbot --nginx -d domain_name.com #replace with your domain name
So that I can be more enthusiastic about writing, please treat me